Video Streaming System Design
Table of content:
- Functional Requirements
- Non Functional Requirements
- Capacity Estimation
- High Level System Design
- Algorithms
YouTube or Netflix are popular video streaming services. These services allow users to upload / stream /share videos.
Functional Requirements
- User should be able to upload and stream videos
- System should support different video formats
- System should recommend videos to viewers
Non-Functional Requirements
- System should highly reliable/scalable
- System should be highly available with eventual consistency
Capacity Estimation
Throughput
- DAU : 5M users and each user watch 5 video/day
- 25Million views/day-> 250 Views/sec(RPS)
- 10% of users upload-> 5 videos/sec
Storage
- 5M X 500MB= 250 TB/day
High Level System Design
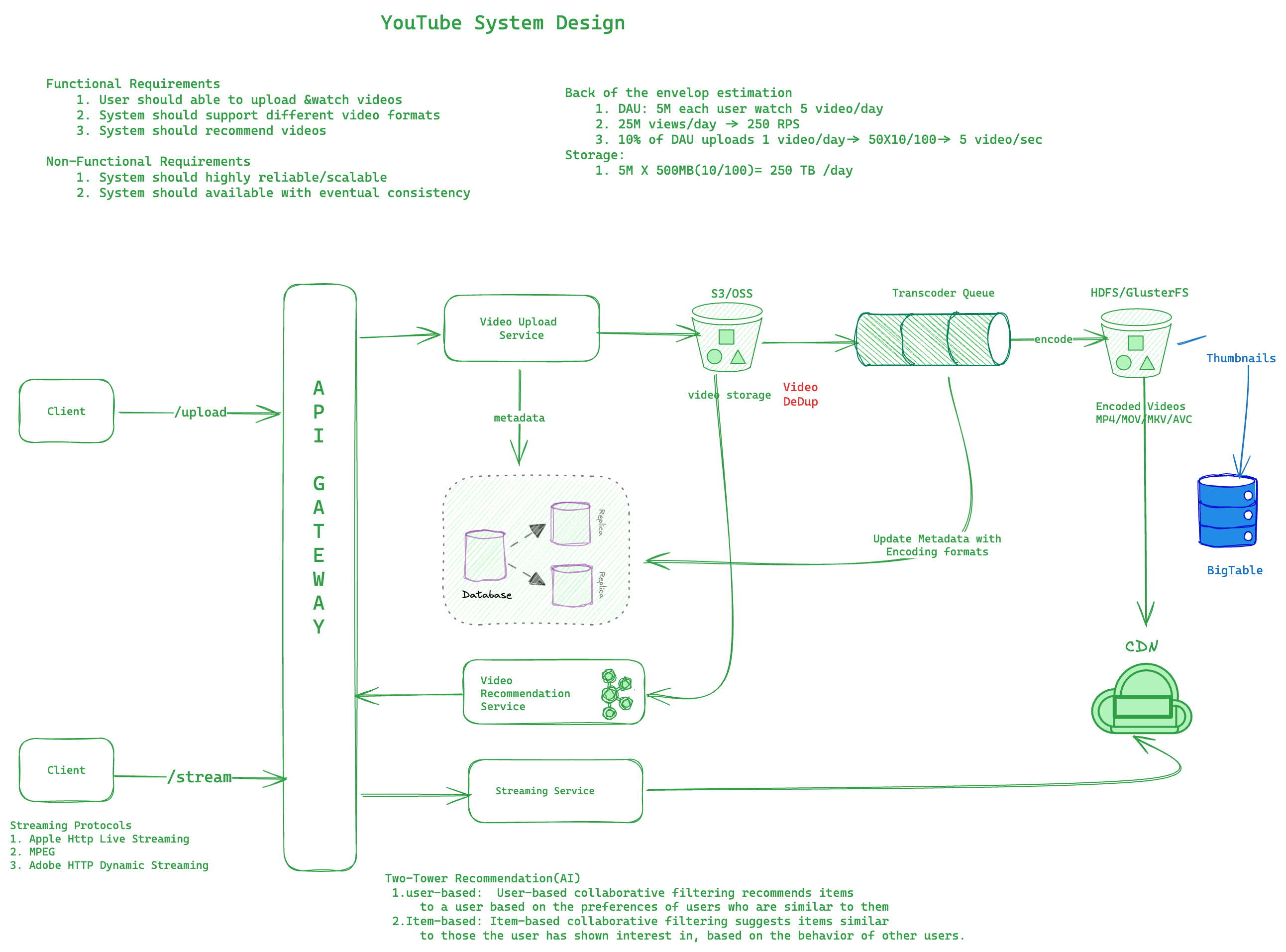
- User uploads videos into object storage and updates medata into database(Cassandra)
- Transcoder service will read from queue process them asynchronously and encodes into different format.
- Different video formats make it available to CDN to improve view experience.
- Video recommendation service uses two-tower approach to generation video recommendations.
- Client stream videos on their devices using different streaming protocols such as Apple HTTP Live/MPEG.
Algorithms
Below algorithms helps to find top K movie recommendation using Heap DataStructure.
from heapq import *
class ListNode:
def __init__(self, val=0, nextNode=None):
self.val= val
self.next = nextNode
def topKMovies(arrays):
heap = []
for head in arrays:
heappush(heap, (head.val, head))
topMovies = []
while heap:
nodeVal, node = heappop(heap)
topMovies.append(nodeVal)
if node.next:
heappush(heap, (node.next.val, node.next))
return topMovies
if __name__=="__main__":
n1 = ListNode(1, ListNode(5, ListNode(7, None)))
n2 = ListNode(2, ListNode(4, ListNode(8, None)))
array = [n1, n2]
print(topKMovies(array))